Sometimes, you may want to get only the private (i.e. non-customer) order notes for an order.
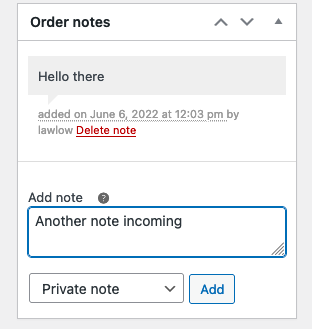
You can use the builtin WooCommerce function wc_get_order_notes
for this. All you have to do is set the ‘type’ query argument to ‘internal’. It will return an array of order notes.
Example snippet
In this example, I want to check the private order notes for a specific text when an order status is changed to ‘processing’.
Let’s say the text string I’m looking for is “This is already completed”. If there is a match, then the order will be automatically marked as completed.
add_action( 'woocommerce_order_status_processing', 'check_private_order_notes', 10, 1); function check_private_order_notes( $order_id ) { // 1. get order notes, assign it to a variable $private_order_notes = wc_get_order_notes([ 'order_id' => $order_id, 'type' => 'internal', // only get private notes ]); // 2. Loop through the notes and look for specific text string foreach( $private_order_notes as $note ) { if( $note->content == 'This is already completed' ) $matching_text = true; } // 3. Update status if there was a match if( $matching_text ) { $order = wc_get_order($order_id); $order->update_status( 'completed' ); } }
Conversely, you can also get only the “Note to customer” notes by setting ‘type’ to ‘customer’.
See all the parameters you can change here: https://woocommerce.github.io/code-reference/namespaces/default.html#function_wc_get_order_notes
Hope this helps someone.
😛